How to code an AI assistant from scratch
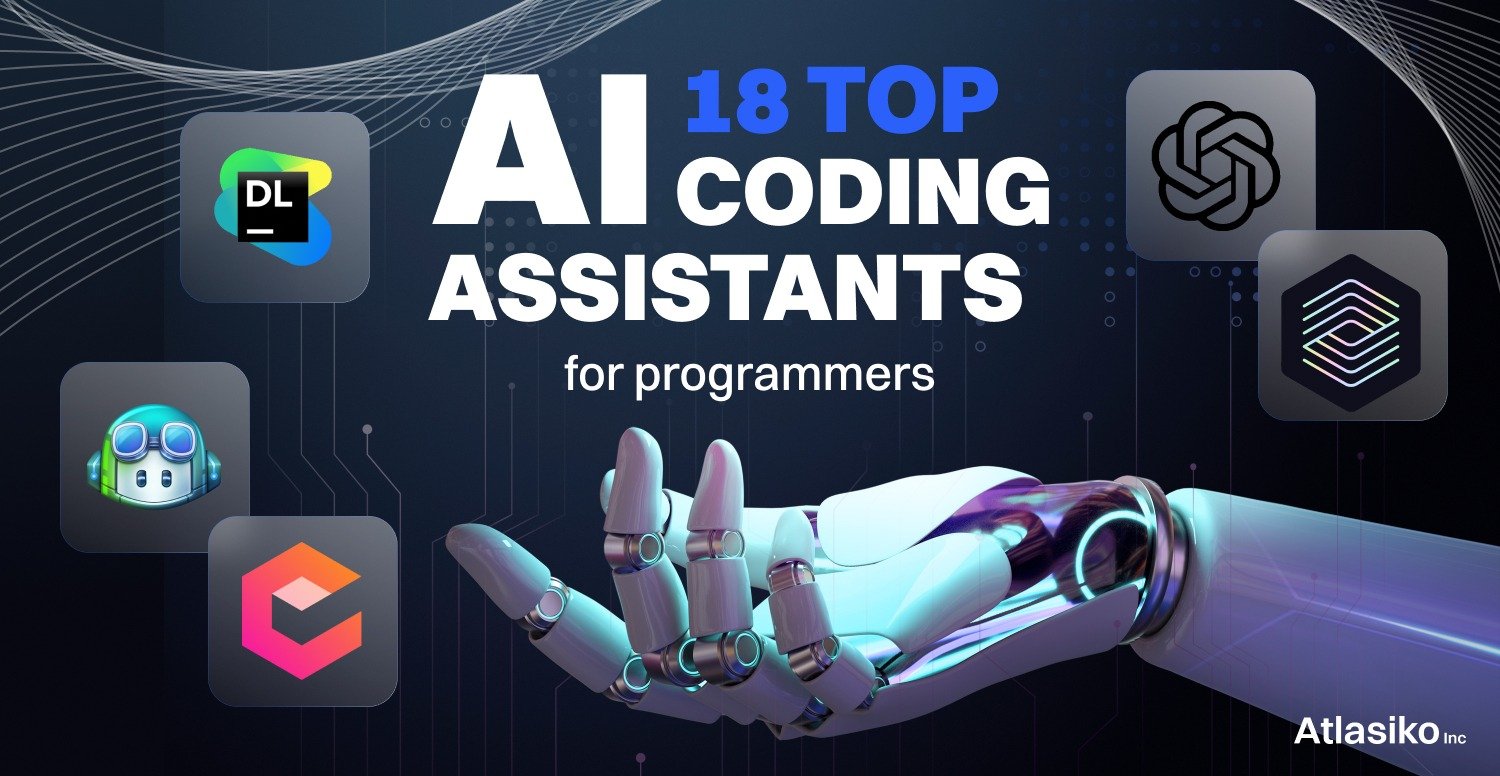
How to Code an AI Assistant from Scratch: A Complete Guide
Building an AI assistant from scratch is an exciting and challenging project that requires a combination of programming, artificial intelligence, and natural language processing (NLP) skills. AI assistants like Jarvis from the Iron Man movies or real-world AI coding assistants such as GitHub Copilot are built using complex algorithms, machine learning models, and automation techniques. If you are wondering how to build a virtual assistant that can help with coding or other tasks, this guide will walk you through the process step by step.
1. Understanding AI Assistants
An AI assistant is a software application that uses artificial intelligence to perform tasks, answer questions, and assist users in various ways. AI assistants can be categorized into:
- Rule-Based Assistants: These follow predefined rules and scripts to respond to queries.
- Machine Learning-Based Assistants: These use deep learning and NLP to improve over time.
- Hybrid Assistants: A combination of rule-based and AI-driven methods for better performance.
2. Defining Your AI Assistant’s Purpose
Before coding an AI assistant, you need to determine its purpose. Will it be a general-purpose assistant like Siri or Google Assistant, or a specialized coding assistant? Some common applications include:
- Personal productivity assistant
- AI-powered coding assistant
- Smart home automation assistant
- Customer service chatbot
Defining the assistant’s role will help you select the right technologies and frameworks.
3. Choosing the Right Technology Stack
Building an AI assistant requires selecting the right technologies. Here’s what you’ll need:
- Programming Language: Python (due to its strong AI and ML libraries)
- Natural Language Processing (NLP): NLTK, spaCy, or Hugging Face Transformers
- Speech Recognition (if voice-based): Google Speech-to-Text, CMU Sphinx
- Machine Learning Frameworks: TensorFlow, PyTorch, OpenAI’s GPT models
- Database for Storing User Data: PostgreSQL, Firebase, MongoDB
- API Integration: OpenAI API, Google APIs, Wolfram Alpha API
- User Interface (Optional): Flask/Django for web apps, React for UI
4. Setting Up the Development Environment
To get started, install the necessary libraries and frameworks. If you’re using Python, set up a virtual environment and install dependencies:
mkdir ai_assistant
cd ai_assistant
python3 -m venv venv
source venv/bin/activate # For Windows: venv\Scripts\activate
pip install openai nltk spacy flask tensorflow speechrecognition
5. Implementing NLP for Your Assistant
Natural Language Processing (NLP) is the backbone of AI assistants. It helps the assistant understand and generate human-like responses. A simple NLP pipeline includes:
- Tokenization (breaking text into words/sentences)
- Lemmatization/Stemming (reducing words to root forms)
- Named Entity Recognition (NER) (identifying names, dates, etc.)
- Intent Recognition (understanding what the user wants)
Here’s an example of text preprocessing with spaCy
:
import spacy
nlp = spacy.load("en_core_web_sm")
doc = nlp("Hello, how can I assist you today?")
for token in doc:
print(token.text, token.lemma_, token.pos_, token.dep_)
6. Adding Speech Recognition and Text-to-Speech
For a voice-based assistant, integrate speech recognition:
import speech_recognition as sr
def recognize_speech():
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
audio = recognizer.listen(source)
try:
text = recognizer.recognize_google(audio)
print("You said: ", text)
return text
except sr.UnknownValueError:
print("Could not understand audio")
return ""
For Text-to-Speech (TTS):
import pyttsx3
def speak(text):
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
7. Integrating AI for Response Generation
You can integrate OpenAI’s GPT model to generate responses dynamically:
import openai
openai.api_key = "your_openai_api_key"
def get_ai_response(prompt):
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": prompt}]
)
return response["choices"][0]["message"]["content"]
8. Building the AI Coding Assistant Component
If your assistant is meant to help with coding, integrate GitHub Copilot API or use OpenAI’s Codex model:
def code_assistant(prompt):
response = openai.ChatCompletion.create(
model="code-davinci-002",
messages=[{"role": "system", "content": "You are a coding assistant."},
{"role": "user", "content": prompt}]
)
return response["choices"][0]["message"]["content"]
You can then call code_assistant("Write a Python function to reverse a string")
to get AI-generated code.
9. Implementing User Interaction (Chatbot UI or CLI)
For a simple CLI-based assistant:
while True:
user_input = input("You: ")
if user_input.lower() in ["exit", "quit"]:
break
response = get_ai_response(user_input)
print("Assistant:", response)
For a web-based UI, use Flask:
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route("/", methods=["GET", "POST"])
def home():
if request.method == "POST":
user_input = request.form["query"]
response = get_ai_response(user_input)
return render_template("index.html", response=response)
return render_template("index.html")
if __name__ == "__main__":
app.run(debug=True)
10. Deploying Your AI Assistant
Once you’ve built your AI assistant, you can deploy it:
- Locally: Run the Python script on your machine.
- Web Server: Deploy using Flask on Heroku or AWS.
- Mobile Integration: Use React Native for mobile interfaces.
- Voice Assistant: Integrate with IoT devices or smart home systems.
Final Thoughts
Building an AI assistant from scratch requires an understanding of NLP, machine learning, APIs, and software development. Whether you want to create a virtual assistant like Jarvis or a coding assistant, the key steps include:
- Defining the assistant’s purpose
- Choosing the right tech stack
- Implementing NLP, speech recognition, and AI models
- Developing a user-friendly interface
- Deploying the assistant for real-world use
With patience and practice, you can build a powerful AI assistant tailored to your needs. Happy coding! image/atlasiko